How to import points from MS Excel in NX?

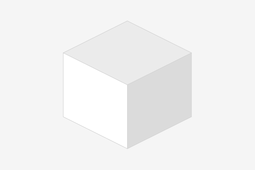
Use these scripts to import 3D coordinates from MS Excel file into NX model (unfortunately sample script and files attachments not allowed).
-
Step 1:
Press ALT+F8 keys, select the file which contains the following code (extension is .vb):
Option Strict Off
Imports System
Imports NXOpen
Module NXJournalSub Main(ByVal args() As String)
Dim theSession As Session = Session.GetSession()
Dim workPart As Part = theSession.Parts.Work()
Dim pnt As Point3d
Dim newPoint As Point
Dim X, Y, Z As Double
Dim EXCEL = CreateObject("Excel.Application")
EXCEL.Visible = False
Dim Doc = EXCEL.Workbooks.Open("C:\Siemens NX\KBE\Points.xlsx", ReadOnly:=True)
Dim Sheets = EXCEL.Sheets
For i As Integer = 1 To Doc.Sheets.Count
Dim Sheet = Doc.Sheets.Item(i)
Dim pointCounter As Integer
For pointCounter = 0 To Sheet.UsedRange.Rows.Count - 1
X = Sheet.Cells((pointCounter + 1), 1).Value
Y = Sheet.Cells((pointCounter + 1), 2).Value
Z = Sheet.Cells((pointCounter + 1), 3).Value
pnt = New Point3d(X, Y, Z)
newPoint = workPart.Points.CreatePoint(pnt)
newPoint.SetVisibility(SmartObject.VisibilityOption.Visible)
Next
workPart.ModelingViews.WorkView.Fit()
Next
Doc.Close()
EXCEL.Quit()
End Sub
End Module -
Step 2:
The Excel file location is in this folder:
"C:\Siemens NX\KBE\Points.xlsx"
Change the folder or location as you desired.The result can be seen on this video:
https://www.youtube.com/watch?v=4V-NsKdTUmY -
Step 3:
Faster method with openfile dialog, press ALT+F8 keys, select the file which contains the following code (extension is .vb):
Option Strict Off
Imports System
Imports NXOpen
Imports System.Windows.FormsModule NXJournal
Sub Main(ByVal args() As String)Dim openFileDialog1 As New OpenFileDialog()
openFileDialog1.Filter = "Excel files (*.xls, *.xlsx)|*.xls;*.xlsx|All files (*.*)|*.*"
openFileDialog1.FilterIndex = 1
If openFileDialog1.ShowDialog() = DialogResult.OK Then
Dim theSession As Session = Session.GetSession()
Dim workPart As Part = theSession.Parts.Work
Dim pointsFromFile As NXOpen.GeometricUtilities.PointsFromFileBuilder
pointsFromFile = workPart.CreatePointsFromFileBuilder()
Dim EXCEL = CreateObject("Excel.Application")
EXCEL.Visible = False
EXCEL.DisplayAlerts = False
Dim Doc = EXCEL.Workbooks.Open(openFileDialog1.FileName, ReadOnly:=True)
Dim fileFolder As String = IO.Path.GetDirectoryName(openFileDialog1.FileName)
Dim Sheets = EXCEL.Sheets
For i As Integer = 1 To Doc.Sheets.Count
Dim Sheet = Doc.Sheets.Item(i)
Dim newCSV As String = fileFolder & "\" & Sheet.Name & ".csv"
Sheet.SaveAs(newCSV, 20, , , , , , 2)
pointsFromFile.FileName = newCSV
pointsFromFile.CoordinateOption = NXOpen.GeometricUtilities.PointsFromFileBuilder.Options.Absolute
pointsFromFile.Commit()
workPart.ModelingViews.WorkView.Fit()
Next
pointsFromFile.Destroy()
Doc.Close()
EXCEL.Quit()
For Each FileDelete As String In IO.Directory.GetFiles(fileFolder & "\", "*.csv")
IO.File.Delete(FileDelete)
Next
End If
End Sub
End ModuleThe result can be seen on this video:
https://www.youtube.com/watch?v=zpZsMIH21iU